Types of Input
text
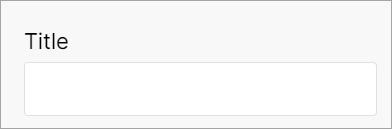
For entering text for name, title, description, etc.
textarea
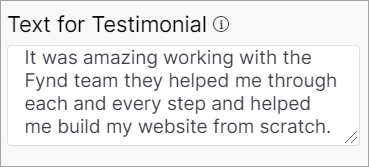
For entering large text spanning a couple of sentences.
checkbox
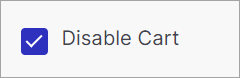
For selecting or opting in.
radio
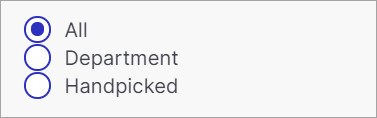
For selecting an option amongst many options.
range
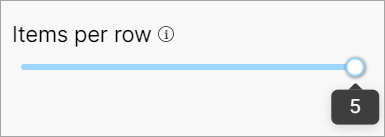
For defining a limit.
select
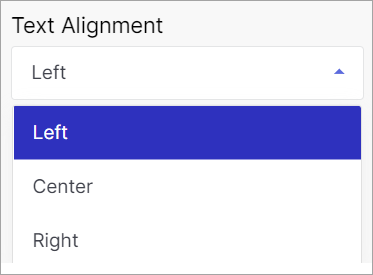
For showing a drop-down of options to choose from.
colour
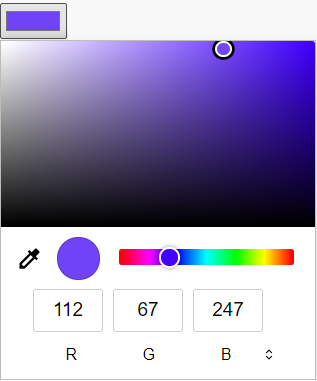
For choosing a colour for text, background, buttons, etc.
code
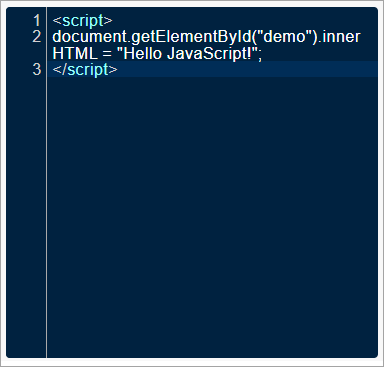
An IDE (code editor) for typing or entering a code.
url
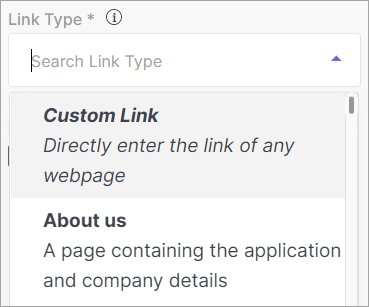
A link builder for pointing to a resource or a URL (web address).
product
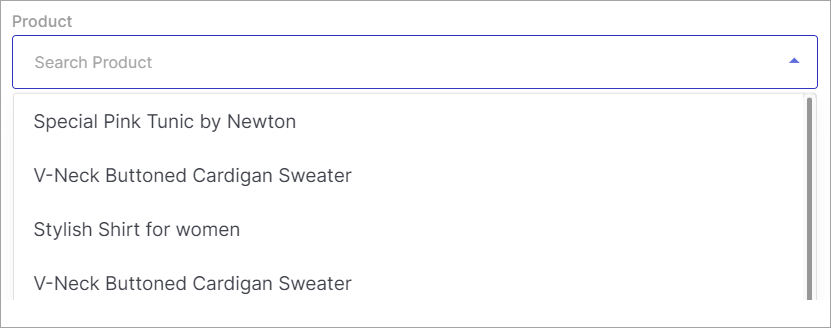
A dropdown with a list of products.
brand
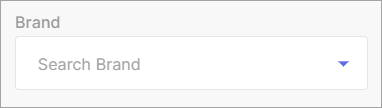
A dropdown with a list of brands.
collection
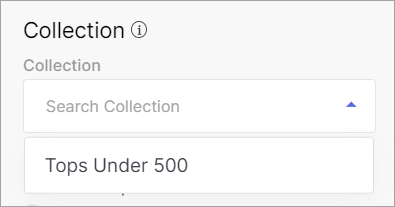
A dropdown with a list of collections.
department
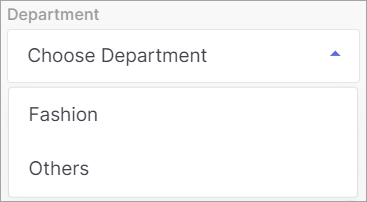
A dropdown with a list of departments.
image
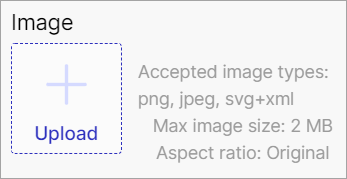
For uploading and selecting an image.
extension
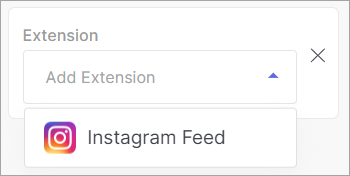
For adding extensions and placing them in the position exposed by the theme.